We will discuss a couple of Errors and One Gotcha related to Python arguments and parameters.
Before moving on, let’s discuss a few terms. Check out the code snippet below. It’s a simple function that prints the sum of its parameters.
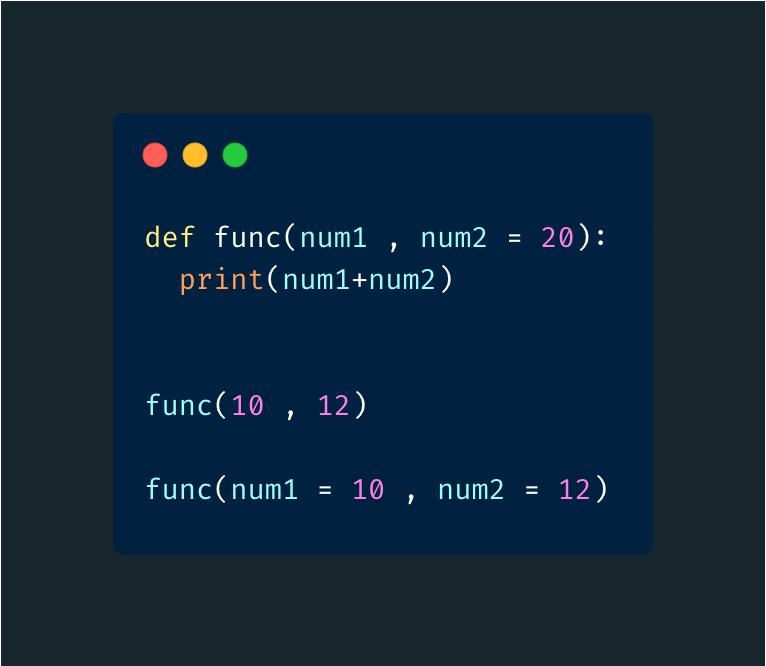
- Parameters- They are the names listed in the function’s definition. Eg: In the above code snippet, num1 and num2 are parameters
- Arguments- They are the values we pass while calling the function. Eg: In the above code snippet, in both calls to the function 10 and 12 are arguments passed to the function
- Positional Argument- When we pass arguments to a function without explicitly assigning them to parameters. Eg: In the above code snippet, we use positional arguments in the first call to func
- Keyword Argument- When we pass arguments to a function by explicitly assigning them to parameters. Eg: In the above code snippet, we use keyword arguments in the second call to func
- Default Values- The value assigned to the parameter during the function definition. Eg: In the above code snippet, 20 is a default value assigned to the parameter num2
Order of default parameters
RULE: If you have a parameter with a default value when defining your function, all the parameters following it MUST also have default values
Incorrect
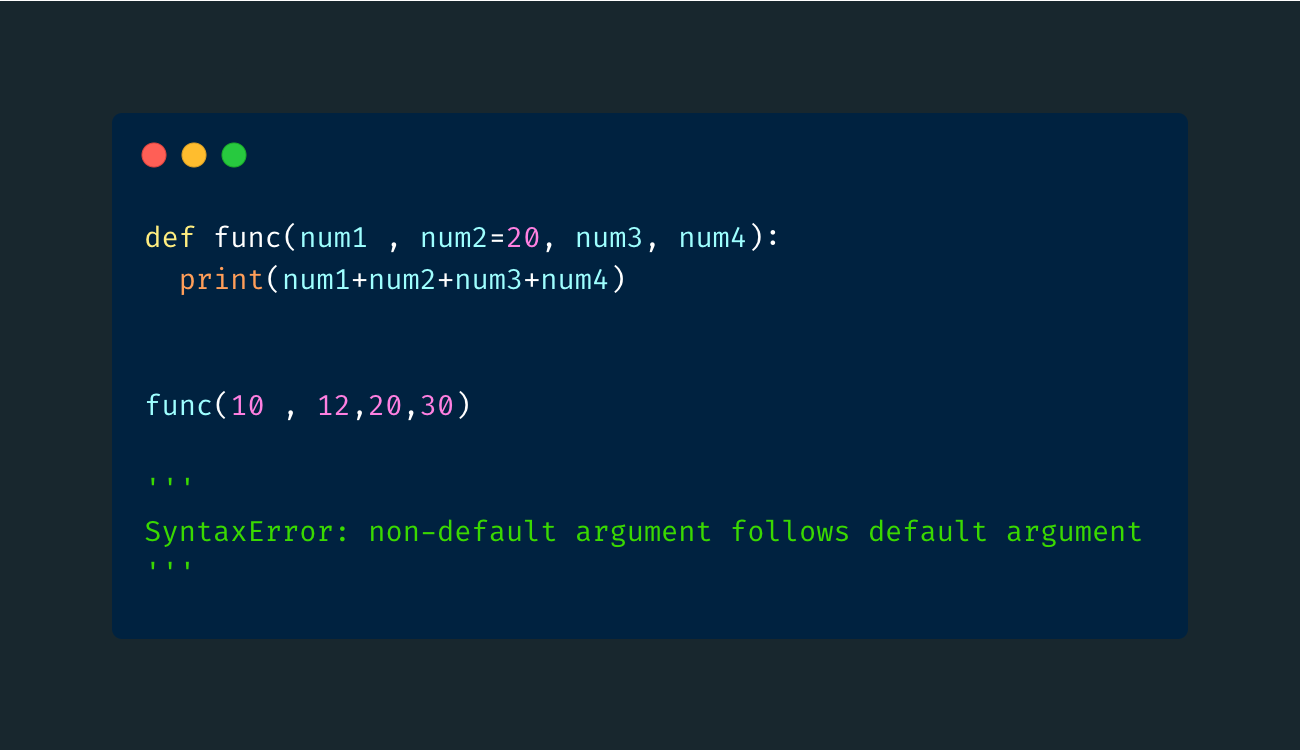
In the above function definition for func, a couple of parameters (num3, num4 )with no default value follow a parameter (num2 )with a default value. This results in a Syntax Error.
Correct
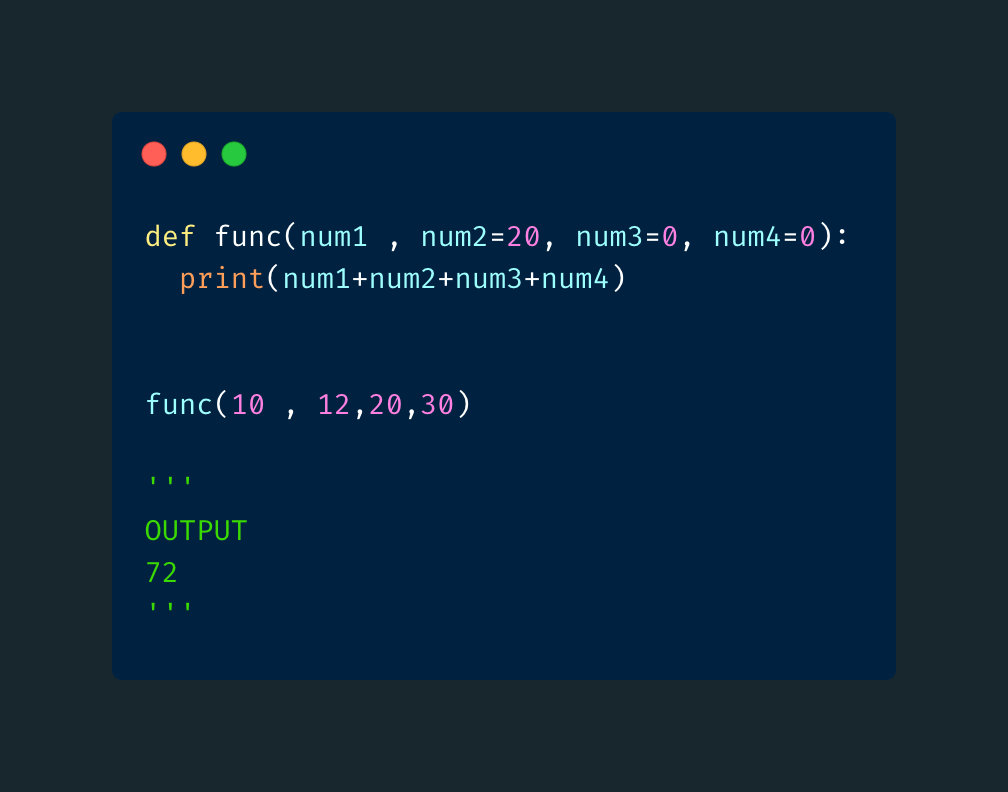
This doesn’t result in any error since we have specified default values for the variables num3 and num4
Order of keyword argument
RULE: When calling a function, a keyword argument must follow a keyword argument. Therefore, No positional arguments can follow a keyword argument.
Incorrect
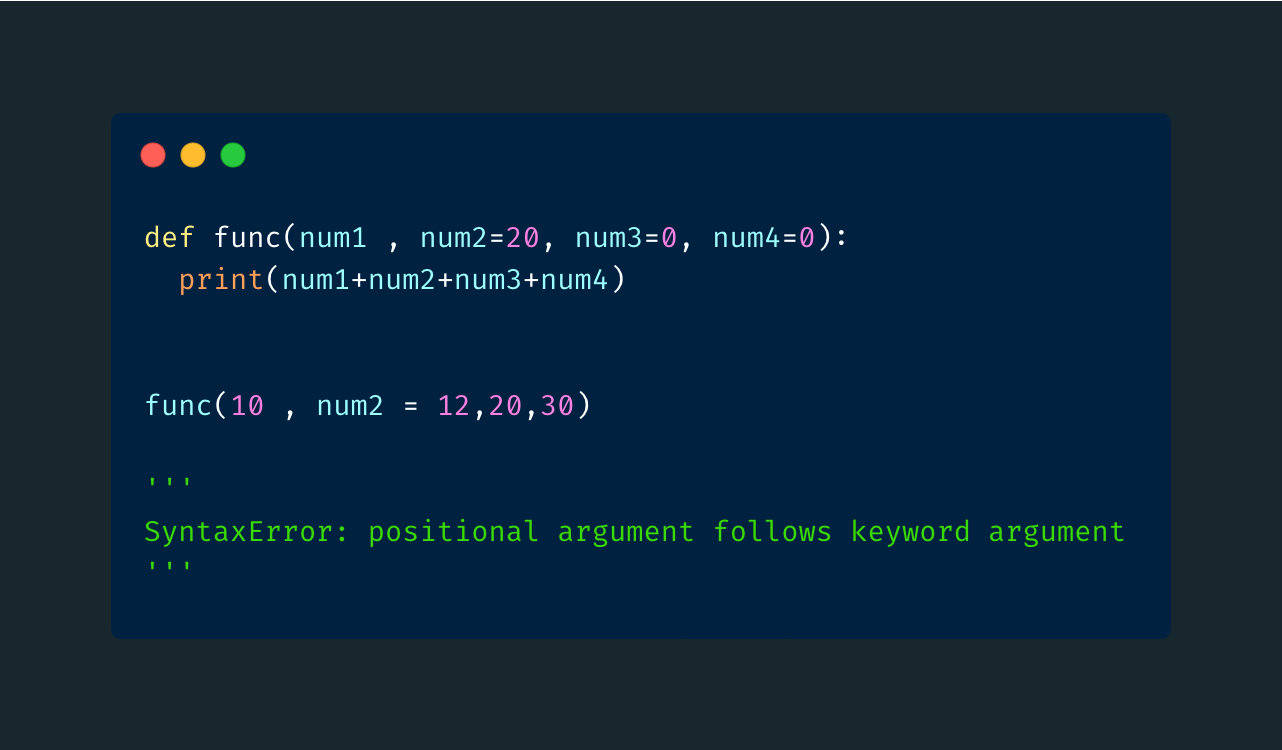
12 is passed as a keyword argument. However, the arguments following it (20 and 30) are passed as positional arguments. This results in a Syntax Error.
Correct
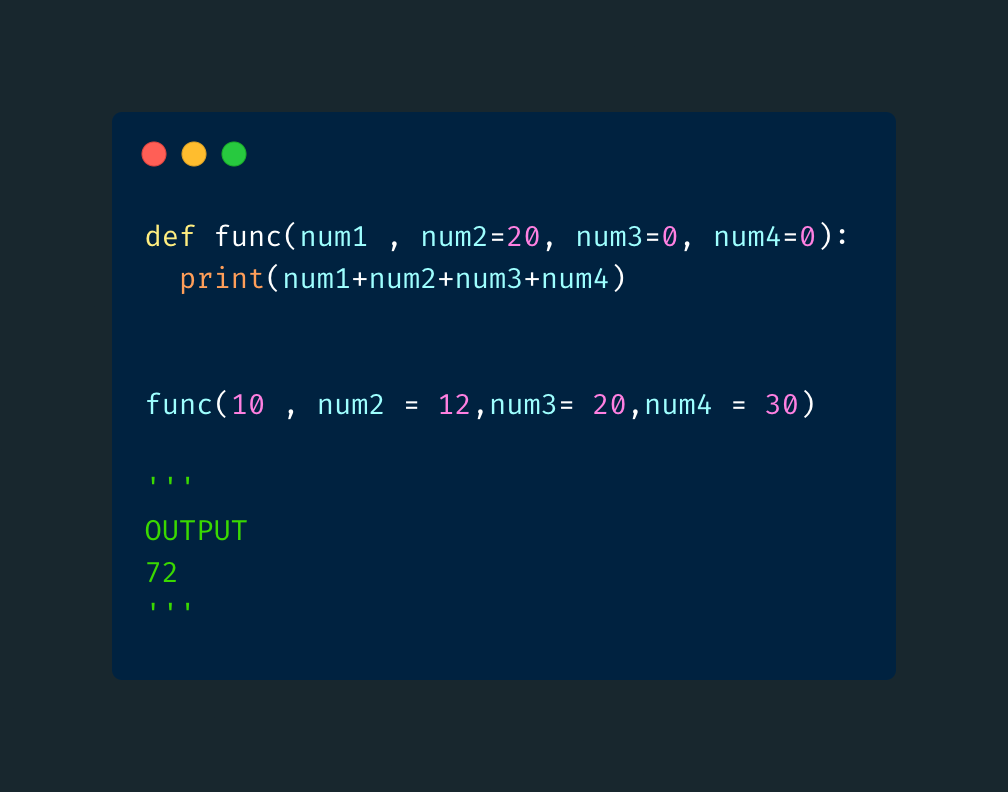
This time, we pass the arguments (20 and 30) as keyword arguments. Therefore, no error is raised.
Value of Default Parameters
MISCONCEPTION: Default Values are assigned to parameters during each function call
Correct: The default value is only assigned once. In the future functions calls the previous value is used
This will make more sense when you follow the example below

The function has two parameters num and arr. arr has a default value of an empty list. The function doesn't do anything fancy. It simply inserts the parameter num into the array arr and then prints the array.
Expectation
''' OUTPUT [10] [20] [30] '''
So basically each time we call the function, arr is set to the default value of an empty list, and num is inserted into it.
But this is NOT the correct output
Actual
''' OUTPUT [10] [10, 20] [10, 20, 30] '''
Huh, weird right?🤯
Basically, the default value is set during the first function call. During the following function calls, the default value is not assigned again. Instead, the previous value is used.
Solution
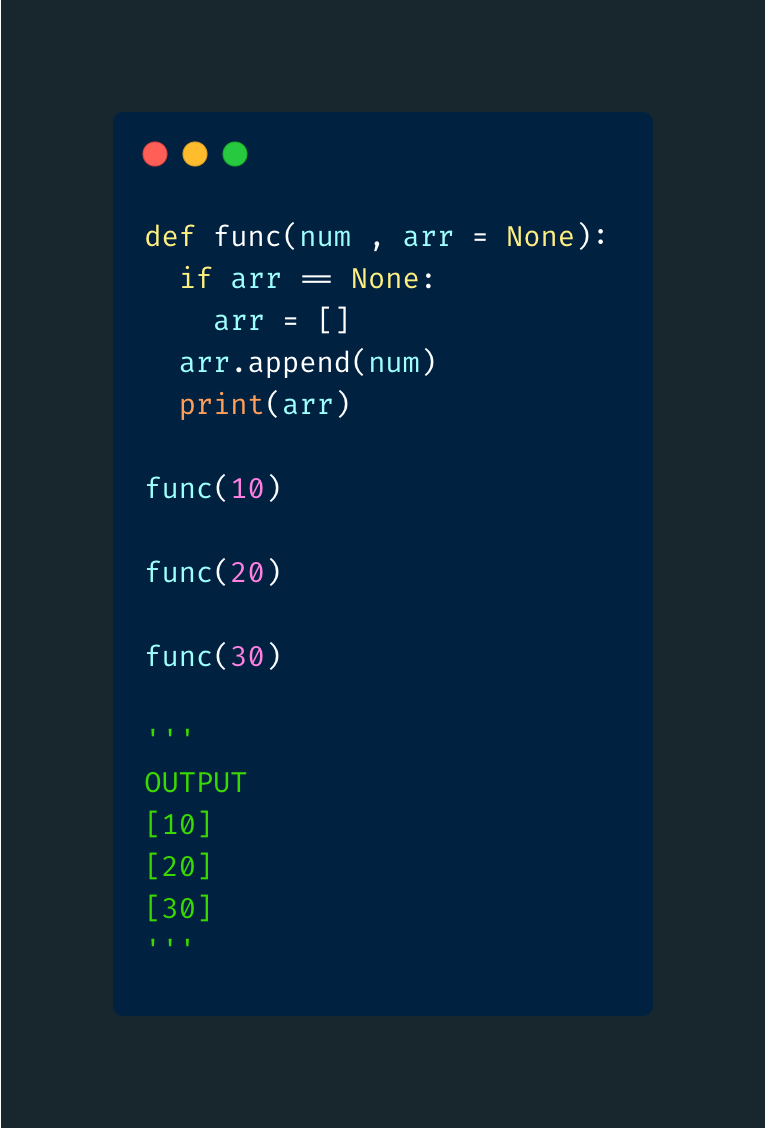
Instead of assigning the empty list as a default parameter, assign None as a default value and assign the empty list inside the function.
Conclusion
I hope you learned something new today. Parameters and Arguments seem like easy concepts but in reality, they are slightly more complex than you think. Now you know the errors and misconceptions to avoid.